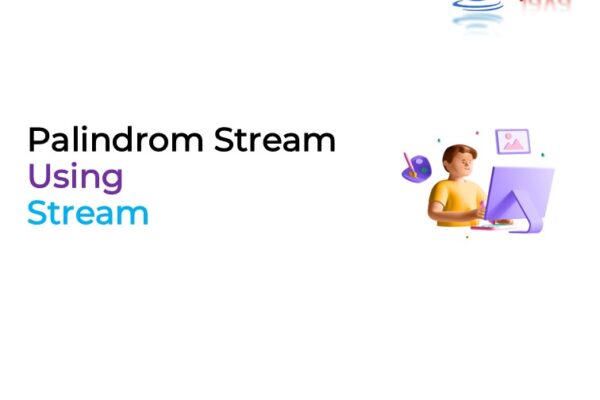
Java Program to Check Palindrome String using stream
In this example, we are going to learn how to find string is palindrome or not using stream. Steps to solve this problem : 1. First we will iterate string by half of its size 2. We will compare each character so here we are comparing ith element with (length-i-1)th element if all matches then…
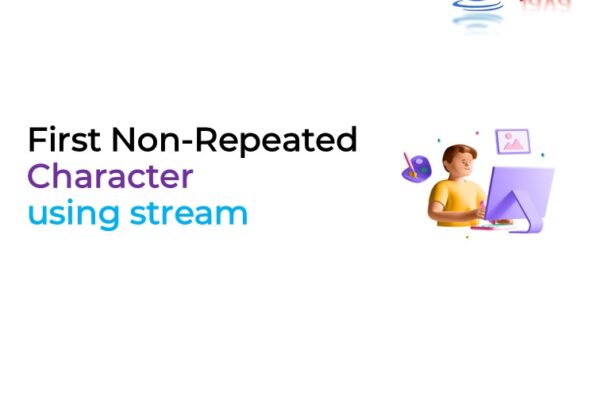
First Non-Repeated Character using stream in java
In this example, we will learn how we can find first non-repeated character using stream so first we will go oversteps how to solve this problem. Step 1: Iterate all the character from input stream Step 2: Now we will count character occurrence and will store this result into LinkedHashMap, why we have used…
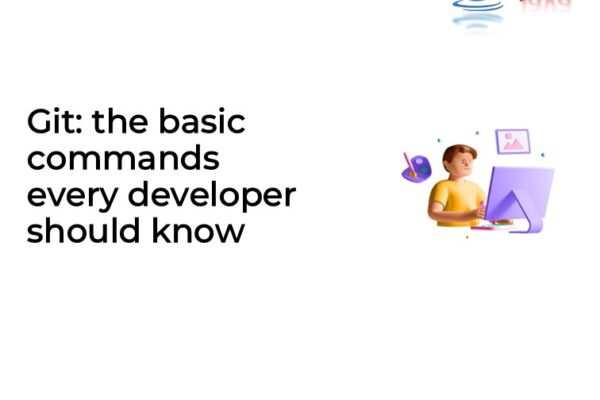
Git: the basic commands every developer should know
In this blog, We are going to discuss basic git commands : 1. git init This command will create a new Git repository in the current directory and it will create a `.git` folder where version control information is stored. 2. git clone <repository> This command will create a local copy of a remote repository…
Java 8 Stream : Map Sum values example using mapToInt
Stream : mapToInt method mapToInt method is introduced in stream and its designed to transform a stream of object into intStream. What is intStream ? IntStream is specialized stream that deals with primitve int value which can be more efficient than regular Stream<Integer> Key Points : 1.What is purpose mapTOInt method ? mapToInt is used…
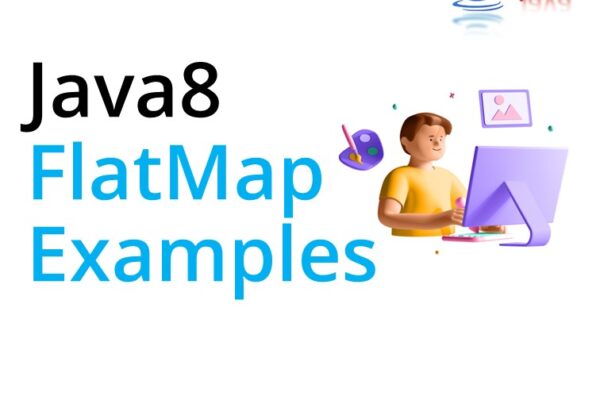
Java 8 Stream : FlatMap example
In this tutorial we are going to learn how to use flatmap. 1. Basic FlatMap Example: Explanation: nestedList is a list of lists. flatMap is used to flatten the nested structure into a single stream of strings. The resulting flatList contains all individual strings from the nested lists. 2. FlatMap with Arrays: Explanation: Stream.of creates…
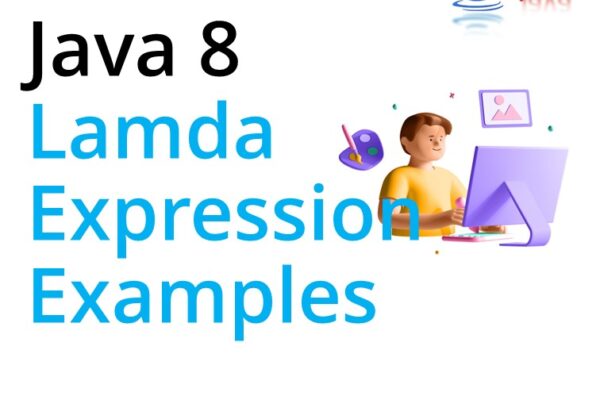
Java 8 Lamda Expression basic example
Basic Example: () -> System.out.println(“Hello, Lambda!”); Lambda expression with no parameters, printing a message. Single Parameter: (x) -> x * x; Lambda with a single parameter, returning the square. Multiple Parameters: (a, b) -> a + b; Lambda with multiple parameters, returning their sum. Functional Interface: Comparator<String> comparator = (s1, s2) -> s1.compareTo(s2); Lambda for…
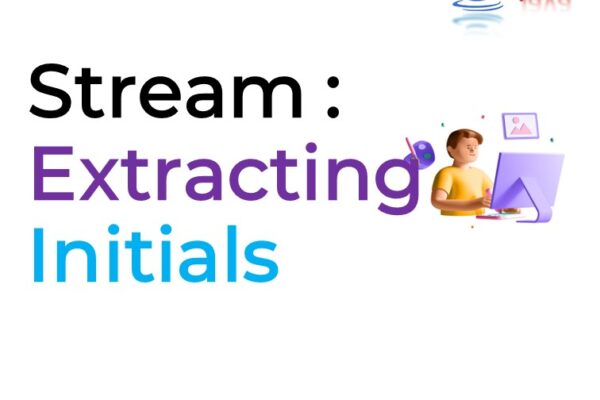
How to extract initials using stream in java?
How to extract initials using stream in java In this Java code snippet, a list of strings named `names` is created, containing full names like “John Doe,” “Jane Smith,” and “Alice Johnson.” Using Java 8 streams, a new list called `initials` is generated by applying a transformation to each full name in the `names` list….
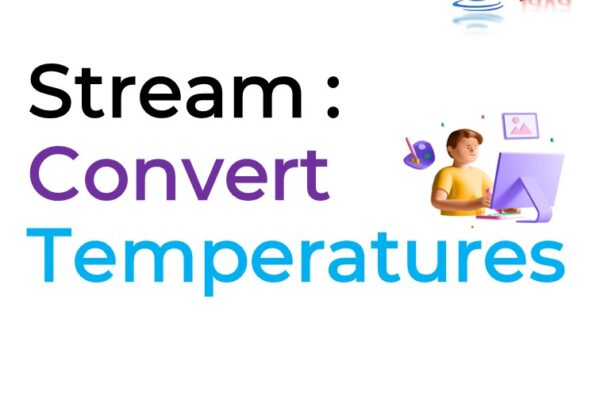
How to Convert temperature using stream in java ?
How to Convert temperature using stream in java In this Java code snippet, a list of double values named `celsiusTemps` is created, containing temperatures in Celsius: 0.0, 10.0, 20.0, and 30.0. Using Java 8 streams, a new list called `fahrenheitTemps` is generated by applying a conversion formula to each Celsius temperature. The `map` operation transforms…
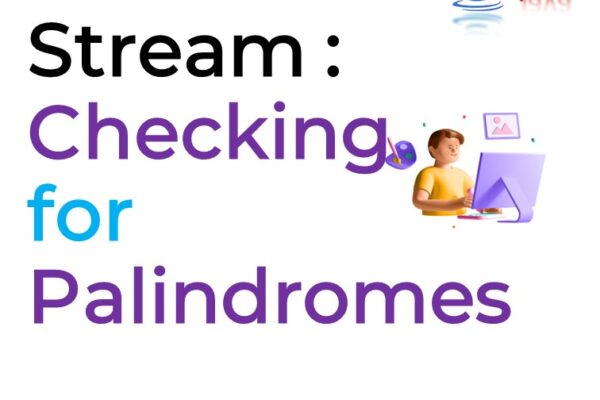
How to check Palindrome in java using Stream API ?
How to check Palindrome in java using Stream API In this Java code snippet, a list of strings named `words` is created, containing “radar,” “hello,” and “deified.” Using Java 8 streams, a new list called `palindromes` is generated by filtering the `words` list. The `filter` operation checks if a string is a palindrome by comparing…
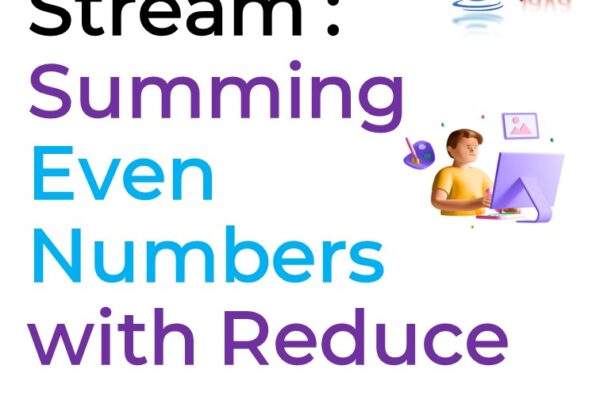
How to sum even numbers with Reduce in java ?
How to sum even numbers with Reduce in java In this Java code snippet, a list of integers named `numbers` is created with values 2, 4, 6, 8, and 10. Using Java 8 streams, the code calculates the sum of even numbers from the `numbers` list. The `filter` operation selects only even integers by checking…